Lets examine the 4x4x4 Hadamard cube in more detail.
Recall from 3D-Hadamard Codes discussion, that a 4x4x4 Hadamard cube is defined as follows:
H_{4} = \begin{bmatrix} \begin{bmatrix} 1&1&1&1 \\ 1&-1&1&-1\\ 1&1&-1&-1\\ 1&-1&-1&1 \end{bmatrix} \begin{bmatrix} -1&1&-1&1 \\ 1&1&1&1\\ -1&1&1&-1\\ 1&1&-1&-1 \end{bmatrix} \begin{bmatrix} -1&-1&1&1\\ -1&1&1&-1\\ 1&1&1&1\\ 1&-1&1&-1 \end{bmatrix} \begin{bmatrix} 1&-1&-1&1\\ -1&-1&1&1\\ -1&1&-1&1\\ 1&1&1&1 \end{bmatrix} \end{bmatrix}
Converting this to a binary Walsh cube, we get the following:
W_{4} = \begin{bmatrix} \begin{bmatrix} 0&0&0&0 \\ 0&1&0&1\\ 0&0&1&1\\ 0&1&1&0 \end{bmatrix} \begin{bmatrix} 1&0&1&0 \\ 0&0&0&0\\ 1&0&0&1\\ 0&0&1&1 \end{bmatrix} \begin{bmatrix} 1&1&0&0\\ 1&0&0&1\\ 0&0&0&0\\ 0&1&0&1 \end{bmatrix} \begin{bmatrix} 0&1&1&0\\ 1&1&0&0\\ 1&0&1&0\\ 0&0&0&0 \end{bmatrix} \end{bmatrix}
Numbering the columns, rows, and faces from 0 to 3 (or using the binary values 00, 01, 10, and 11), we can rearrange the columns, rows, and faces to create a Karnaugh map. This is similar to what was done in the Fast Code Character Generation discussion, but this time we need to account for the z axis.

This results in the following relationship for each value of Z:
z | f(x_0,y_0,x_1,y_1) |
00 | (x_0 \bullet y_0) \oplus (x_1 \bullet y_1) |
01 | (\overline x_0 \bullet \overline y_0) \oplus (x_1 \bullet y_1) |
10 | (x_0 \bullet y_0) \oplus (\overline x_1 \bullet \overline y_1) |
11 | (\overline x_0 \bullet \overline y_0) \oplus (\overline x_1 \bullet \overline y_1) |
Notice that when z_0 is 1, x_0 and y_0 are inverted. Also, when z_1 is 1, x_1 and y_1 are inverted.
The truth table below shows that this is simply a exclusive or relationship:
z | x | output |
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
This allows us to combine the four functions from Table 1 as follows:
f(x_0,y_0,z_0,x_1,y_1,z_1) = ((x_0 \oplus z_0) \bullet (y_0 \oplus z_0)) \oplus ((x_1 \oplus z_1) \bullet (y_1 \oplus z_1))
This can be written more generically to work with any Walsh cube of size 2^n .
f(x_0,y_0,z_0, ... , x_n,y_n,z_n) = ((x_0 \oplus z_0) \bullet (y_0 \oplus z_0)) \oplus ... \oplus((x_n \oplus z_n) \bullet (y_n \oplus z_n))
In the above function, the x and y terms are always XORed with a z term. We can construct two additional variants of this function. One where the x a z terms are XORed with a y term and another where the y and z terms are XORed with an x term. All three functions produce the same cube, however each one will construct the cube in a different orientation.
f_1(x_0,y_0,z_0, ... , x_n,y_n,z_n) = ((x_0 \oplus z_0) \bullet (y_0 \oplus z_0)) \oplus ... \oplus((x_n \oplus z_n) \bullet (y_n \oplus z_n))\\ f_2(x_0,y_0,z_0, ... , x_n,y_n,z_n) = ((x_0 \oplus y_0) \bullet (y_0 \oplus z_0)) \oplus ... \oplus((x_n \oplus y_n) \bullet (y_n \oplus z_n))\\ f_3(x_0,y_0,z_0, ... , x_n,y_n,z_n) = ((x_0 \oplus y_0) \bullet (x_0 \oplus z_0)) \oplus ... \oplus((x_n \oplus y_n) \bullet (x_n \oplus z_n))
3D Hadamard Cube Variant
Recall from 3D-Hadamard Codes discussion, that the 4x4x4 Hadamard cube variant is defined as follows:
H_{4}^{alt} = \begin{bmatrix} \begin{bmatrix} 1&1&1&1\\ 1&-1&1&-1\\ 1&1&-1&-1\\ 1&-1&-1&1 \end{bmatrix} \begin{bmatrix} 1&-1&1&-1\\ -1&-1&-1&-1\\ 1&-1&-1&1\\ -1&-1&1&1 \end{bmatrix} \begin{bmatrix} 1&1&-1&-1\\ 1&-1&-1&1\\ -1&-1&-1&-1\\ -1&1&-1&1 \end{bmatrix} \begin{bmatrix} 1&-1&-1&1\\ -1&-1&1&1\\ -1&1&-1&1\\ 1&1&1&1 \end{bmatrix} \end{bmatrix}
Converting this to a binary Walsh cube, we get the following:
W_{4}^{alt} = \begin{bmatrix} \begin{bmatrix} 0&0&0&0 \\ 0&1&0&1\\ 0&0&1&1\\ 0&1&1&0 \end{bmatrix} \begin{bmatrix} 0&1&0&1 \\ 1&1&1&1\\ 0&1&1&0\\ 1&1&0&0 \end{bmatrix} \begin{bmatrix} 0&0&1&1\\ 0&1&1&0\\ 1&1&1&1\\ 1&0&1&0 \end{bmatrix} \begin{bmatrix} 0&1&1&0\\ 1&1&0&0\\ 1&0&1&0\\ 0&0&0&0 \end{bmatrix} \end{bmatrix}
Again, numbering the columns, rows, and faces from 0 to 3 (or using the binary values 00, 01, 10, and 11), we can rearrange the columns, rows, and faces to create a Karnaugh map. This is similar to what was done in the Fast Code Character Generation discussion, but this time accounting for the z axis.

This results in the following relationship for each value of Z:
z | f(x_0,y_0,x_1,y_1) |
00 | (x_0 \bullet y_0) \oplus (x_1 \bullet y_1) |
01 | (\overline{\overline x_0 \bullet \overline y_0}) \oplus (x_1 \bullet y_1) |
10 | (x_0 \bullet y_0) \oplus (\overline{\overline x_1 \bullet \overline y_1}) |
11 | (\overline x_0 \bullet \overline y_0) \oplus (\overline x_1 \bullet \overline y_1) |
However, the following truth table shows that a\oplus b is equal to \overline a \oplus \overline b
a | b | \overline a | \overline b | a \oplus b | \overline a \oplus \overline b |
0 | 0 | 1 | 1 | 0 | 0 |
0 | 1 | 1 | 0 | 1 | 1 |
1 | 0 | 0 | 1 | 1 | 1 |
1 | 1 | 0 | 0 | 0 | 0 |
From this, we can re-write the z=11 entry of Table 2 as follows:
(\overline{\overline x_0 \bullet \overline y_0}) \oplus (\overline{\overline x_1 \bullet \overline y_1})
Taking this into account and applying DeMorgan’s Theorem, the relationships from Table 2 can be rewritten as shown in Table 3.
z | f(x_0,y_0,x_1,y_1) |
00 | (x_0 \bullet y_0) \oplus (x_1 \bullet y_1) |
01 | (x_0 + y_0) \oplus (x_1 \bullet y_1) |
10 | (x_0 \bullet y_0) \oplus (x_1 + y_1) |
11 | (x_0 + y_0) \oplus (x_1 + y_1) |
Notice that when z_0 is 1, x_0 and y_0 are ORed rather than ANDed. Also, when z_1 is 1, x_1 and y_1 are ORed rather than ANDed. Another way of describing this relationship is:
((x+y)\bullet z)+((x\bullet y)\bullet \overline z) \\ (x\bullet z) + (y\bullet z) + (x\bullet y\bullet \overline z)
However if x and y are both 1, then it does not matter if z is set or cleared so the above can be simplified to be:
(x\bullet z) + (y\bullet z) + (x\bullet y)
This relationship allows us to combine the four functions from Table 3 as follows:
f(x_0,y_0,z_0,x_1,y_1,z_1) = ((x_0\bullet z_0) + (y_0\bullet z_0) + (x_0\bullet y_0)) \oplus ((x_1\bullet z_1) + (y_1\bullet z_1) + (x_1\bullet y_1))
Finally, this can be written more generically to work with any Alternate Walsh cube of size 2^n .
f(x_0,y_0,z_0, ... ,x_n,y_n,z_n) = ((x_0\bullet z_0) + (y_0\bullet z_0) + (x_0\bullet y_0)) \oplus ... \oplus ((x_n\bullet z_n) + (y_n\bullet z_n) + (x_n\bullet y_n))
Unlike the function found in the previous section, this function already contains equal representation of all terms. So there are no other variations of this function.
Scripts to generate and then print/plot the 3D code sequences, using the functions discussed above, can be found on GitHub. An example plot of a third order Hadamard cube generated by these scrips is shown below.
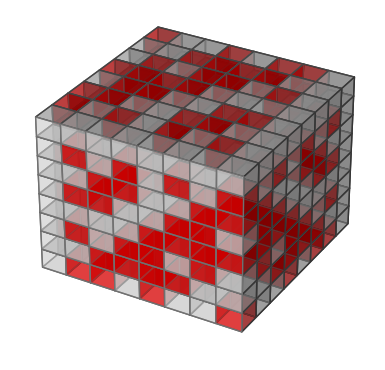